Python字符串小技巧
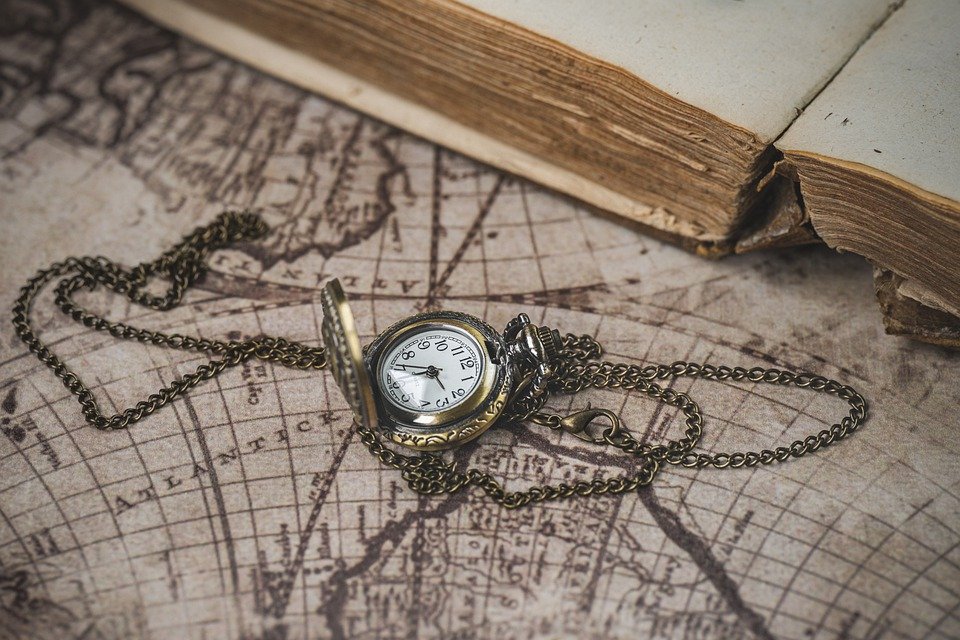
python字符串是最常用的功能,无论是办公自动化还是变成代码,字符串都是必不可少的技能。
本文将介绍python字符串的常用功能、转移字符。
Python的转义字符
Python 转义字符列表我们将在这里介绍三个实际的转义字符用例, 如果你想了解更多关于转义字符的信息,可以在此处(https://python-reference.readthedocs.io/en/latest/docs/str/escapes.html)找到完整的转义字符列表。
- \n – 换行
- \ – 文字反斜杠
- ‘ – 单引号
- \” – 双引号
换行符
有时我们可能想要打印多行字符串,我们可以通过使用单个打印语句结合换行符 \n 将字符串分成多行来实现这一点。
1 | print("this is a long sentence\nand we need to print it\non multiple lines!") |
单引号和双引号
在字符串中使用引号的一种方法是对字符串和实际的引号部分使用不同的引号。如下例所示,如果我们对字符串使用单引号,那么我们将不得不对引号使用双引号,反之亦然。
1 | print('he say, "hello world"') |
字符串常用函数
split()
看单词意思就可以知道,这个方法是用来分割字符串的,它接受参数,参数就是用来分割的标识,默认是空格分割,看个代码:
1 | "abc bcd" s = |
strip()
这个函数用来去掉字符串的空格,它还有两个兄弟函数,rstrip(),lstrip()分别是去掉字符串右边空格和去掉左边空格,代码如下:
1 | " abc " s = |
replace()
这个函数可以进行字符串的替换,接收2个参数,一个是要被替换的串和替换完的串,
1 | "I'm zhangsan" s = |
join()
这个函数可以将列表拼接成一个字符串的形式,
1 | 'a','b','c','d'] mylist = [ |
引号不写内容,默认直接将字符拼接到一起,如果写上分割字符,比如逗号,拼接起来后是这样的:
1 | ",".join(mylist) s = |
startswith(),endswith()
这2个方法经常用在条件判断中, 来判断字符串是否以某个字符开头或以某个字符结尾,举例:
1 | str = "hello, python" |
同理,可以判断字符串以某个字符结尾:
1 | if str.endswith("n"): |
upper(),lower()
upper可以将字符串转成大写,lower将字符串转成小写,这个2个方法一般用带字符判断前,进行字符串转换,转换完毕后再进行判断,比如用户输入的字符,输入Y还是y都可以,你就需要将这些输入后台都转成小写然后进行后续代码的执行:
1 | str = "Hello,Python!!" |